在實作Python網頁爬蟲時,有一個非常重要的議題,就是如何將爬取到的資料有效儲存起來,這時候除了能夠像[Scrapy教學6]解析如何在Scrapy框架存入資料到MySQL教學文章一樣,存入資料庫外,另一個最常使用的方法,就是檔案的匯出,像是CSV、JSON及XML等。
Scrapy網頁爬蟲框架當然也提供了相應的功能,幫助開發人員能夠輕鬆的實作,本文將延續[Scrapy教學6]解析如何在Scrapy框架存入資料到MySQL教學文章,以匯出CSV檔案為例,來和大家分享Scrapy網頁爬蟲框架所提供的兩種檔案匯出方法,包含:
- Scrapy CsvItemExporter(CSV資料模型匯出器)
- Scrapy Feed exports(檔案匯出)
一、Scrapy CsvItemExporter(CSV資料模型匯出器)
第一個方法就是和[Scrapy教學6]解析如何在Scrapy框架存入資料到MySQL教學文章一樣,使用Scrapy網頁爬蟲框架的資料模型管道模組(pipeline)來客製化開發CSV檔案的匯出流程。
來回顧一下目前所建立的「資料模型檔案(items.py)」,如下範例:
import scrapy class NewsScraperItem(scrapy.Item): # define the fields for your item here like: # name = scrapy.Field() post_title = scrapy.Field() post_date = scrapy.Field() post_author = scrapy.Field()
在Scrapy網頁爬蟲(spiders / inside.py)取得資料後,這三個欄位就是後續想要進行資料處理或儲存的欄位,換句話說,也就是「資料模型管道檔案(pipeline.py)」中會使用到的。
開啟「資料模型管道檔案(pipeline.py)」,由於本文想要將爬取到的資料匯出到CSV檔案中,所以就需要引用CsvItemExporter(CSV資料模型匯出器),如下範例:
# Define your item pipelines here # # Don't forget to add your pipeline to the ITEM_PIPELINES setting # See: https://docs.scrapy.org/en/latest/topics/item-pipeline.html # useful for handling different item types with a single interface from itemadapter import ItemAdapter from scrapy.exporters import CsvItemExporter
接下來,新增一個CsvPipeline類別(Class),用來定義Scrapy網頁爬蟲取得的資料匯出到CSV檔案的流程,這個類別名稱可以自行命名,如下範例:
# Define your item pipelines here # # Don't forget to add your pipeline to the ITEM_PIPELINES setting # See: https://docs.scrapy.org/en/latest/topics/item-pipeline.html # useful for handling different item types with a single interface from itemadapter import ItemAdapter from scrapy.exporters import CsvItemExporter class CsvPipeline:
類別(Class)命名完成後,新增一個建構式(Constructor),用來定義初始化的動作,如下範例:
# Define your item pipelines here # # Don't forget to add your pipeline to the ITEM_PIPELINES setting # See: https://docs.scrapy.org/en/latest/topics/item-pipeline.html # useful for handling different item types with a single interface from itemadapter import ItemAdapter from scrapy.exporters import CsvItemExporter class CsvPipeline: def __init__(self): self.file = open('posts.csv', 'wb') self.exporter = CsvItemExporter(self.file, encoding='big5') self.exporter.start_exporting()
以上的初始化動作包含了:
- 建立或打開CSV檔案,設定寫入二進位碼模式(wb, write binary)。
- 建立Scrapy框架的CsvItemExporter(CSV資料模型匯出器)物件,傳入檔案物件及編碼方式,預設為utf-8,如果讀者在匯出CSV檔案後,想要使用Microsoft Excel軟體開啟的話,就需要設定為big5,否則會出現亂碼。
- 呼叫start_exporting()方法(Method)開始進行檔案匯出的動作。
接下來,資料處理的部分,就需要實作Scrapy框架內建的process_item()方法(Method),在其中把資料模型(items)所裝載的資料,透過export_item()方法(Method)傳入CsvItemExporter(CSV資料模型匯出器),如下範例:
# Define your item pipelines here # # Don't forget to add your pipeline to the ITEM_PIPELINES setting # See: https://docs.scrapy.org/en/latest/topics/item-pipeline.html # useful for handling different item types with a single interface from itemadapter import ItemAdapter from scrapy.exporters import CsvItemExporter class CsvPipeline: def __init__(self): self.file = open('posts.csv', 'wb') self.exporter = CsvItemExporter(self.file, encoding='big5') self.exporter.start_exporting() def process_item(self, item, spider): self.exporter.export_item(item) return item
將Scrapy網頁爬蟲取得的資料匯出到自訂的CSV檔案後,結束時所要進行的動作,就要實作內建的close_spider()方法(Method),如下範例:
# Define your item pipelines here # # Don't forget to add your pipeline to the ITEM_PIPELINES setting # See: https://docs.scrapy.org/en/latest/topics/item-pipeline.html # useful for handling different item types with a single interface from itemadapter import ItemAdapter from scrapy.exporters import CsvItemExporter class CsvPipeline: def __init__(self): self.file = open('posts.csv', 'wb') self.exporter = CsvItemExporter(self.file, encoding='big5') self.exporter.start_exporting() def process_item(self, item, spider): self.exporter.export_item(item) return item def close_spider(self, spider): self.exporter.finish_exporting() self.file.close()
以上範例也就是在Scrapy網頁爬蟲結束時,呼叫finish_exporting()方法(Method)完成檔案匯出,並且將檔案物件關閉,釋放資源。
匯出CSV檔案的資料模型管道(pipeline)完成後,別忘了在settings.py檔案,將此資料模型管道(pipeline)加入到ITEM_PIPELINES設定中,如下範例:
# Configure item pipelines # See https://docs.scrapy.org/en/latest/topics/item-pipeline.html ITEM_PIPELINES = { 'news_scraper.pipelines.CsvPipeline': 500, }
最後,利用以下指令來執行Scrapy網頁爬蟲:
$ scrapy crawl inside
執行後會在Scrapy網頁爬蟲專案中,看到posts.csv檔案,利用Microsoft Excel軟體開啟,如下圖:
二、Scrapy Feed exports(檔案匯出)
除了以上在Scrapy網頁爬蟲框架中,利用自訂的CsvItemExporter(CSV資料模型匯出器)來匯出CSV檔案外,Scrapy網頁爬蟲框架也另外提供了簡單的設定方法,即可把爬取到的資料匯出到不同的檔案中。
這個方法非常簡單,剛剛的程式碼及settings.py檔案中的設定完全都不用寫,只要網頁爬蟲(spiders / inside.py)及資料模型檔案(item.py)完成後,直接利用以下的指令就能夠匯出CSV檔案:
$ scrapy crawl inside -o posts.csv
(PS.讀者在執行此方法時,一定要將剛剛自訂的CsvPipeline類別及settings.py檔案中的ITEM_PIPELINES設定註解,才不會發生重複匯出資料的問題)。
目前Scrapy網頁爬蟲框架所支援的檔案有CSV、XML、JSON及JSON lines,只要在指令中更換副檔名即可,是不是非常的方便阿。
當然有時候會有一些特殊的需求,像是編碼及寫入的欄位等,則可以在settings.py檔案中設定,如下範例:
FEED_EXPORT_ENCODING = 'big5' FEED_EXPORT_FIELDS = ['post_title', 'post_date', 'post_author']
特別注意FEED_EXPORT_FIELDS一定要是資料模型檔案(item.py)中所定義的。設定好後,同樣使用剛剛的指令,就能夠匯出Scrapy網頁爬蟲框架所支援的檔案,提升了不少開發效率,而更多的Scrapy Feed exports(檔案匯出)設定屬性可以參考官網文件。
三、小結
本文以匯出CSV檔案為例,分享了Scrapy網頁爬蟲框架所提供的檔案匯出功能,一種就是透過自行開發資料模型管道(pipeline),來客製化定義資料處理所要執行的流程邏輯。
而另一種方法,則是在settings.py檔案中,利用內建的設定屬性,即可方便的透過指令,來匯出相應的檔案類型,適用於沒有特殊或複雜的匯出資料邏輯,來提升資料處理的效率。希望本文能夠幫助大家對Scrapy網頁爬蟲框架的檔案匯出功能有基本的認識。
如果您喜歡我的文章,請幫我按五下Like(使用Google或Facebook帳號免費註冊),支持我創作教學文章,回饋由LikeCoin基金會出資,完全不會花到錢,感謝大家。
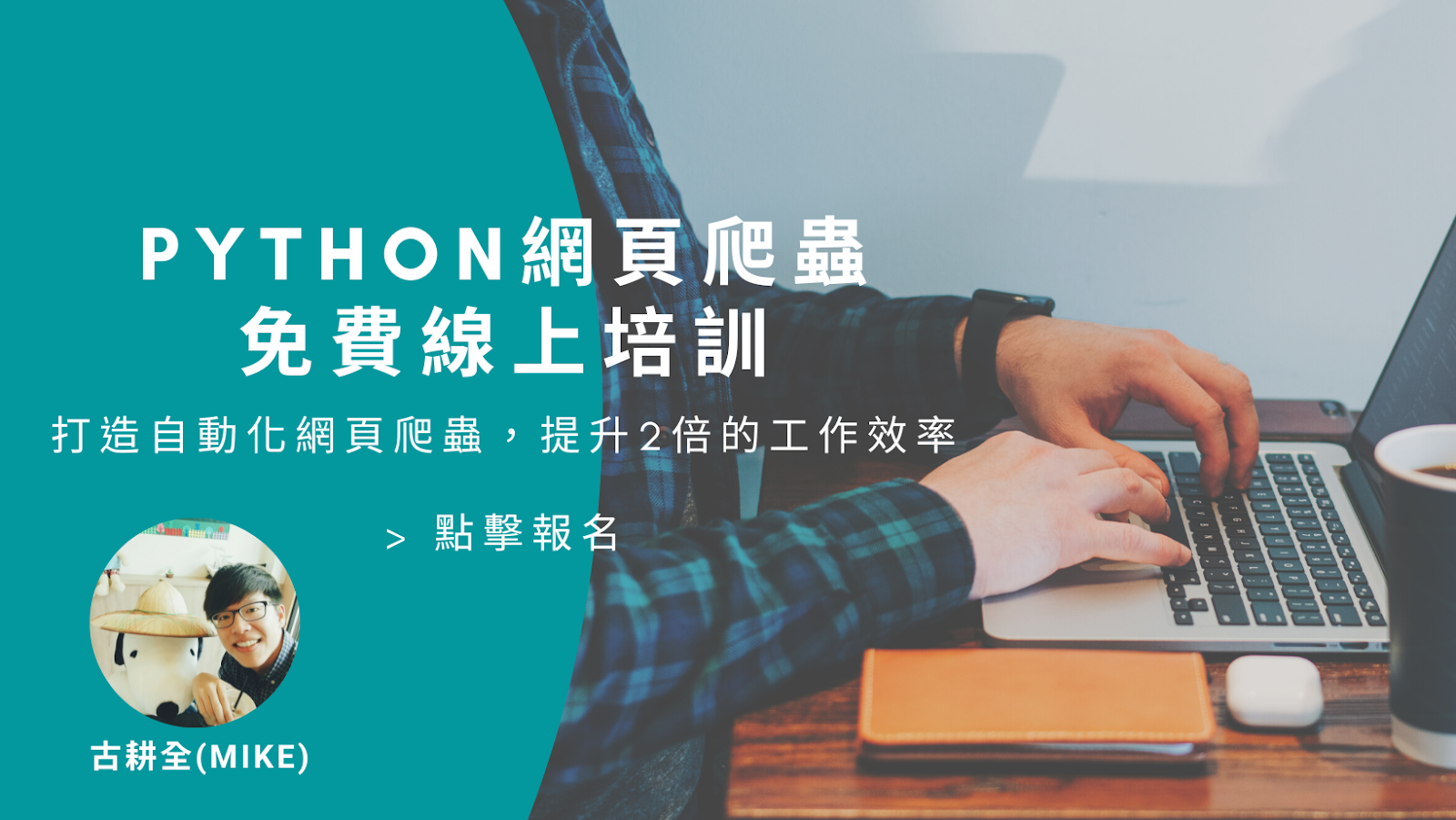
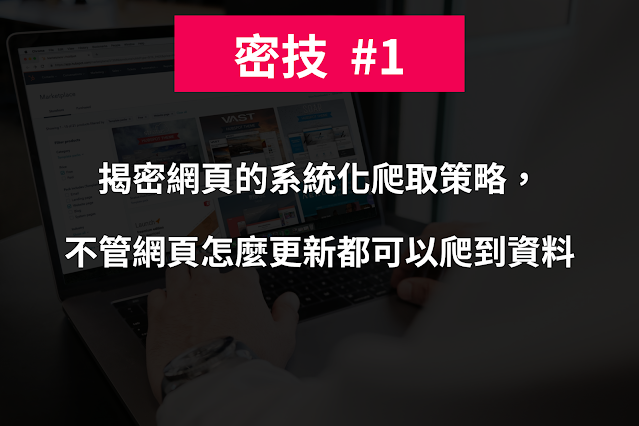
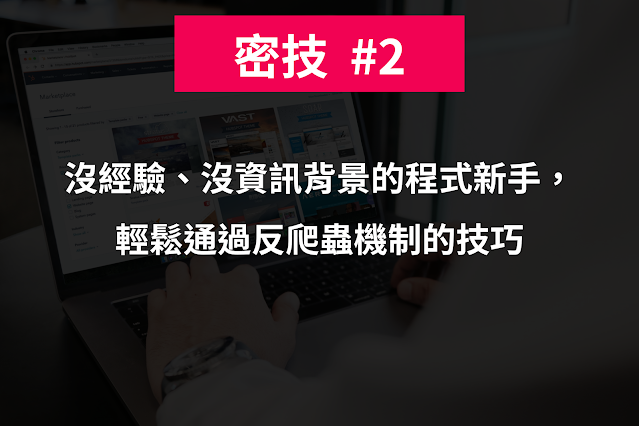
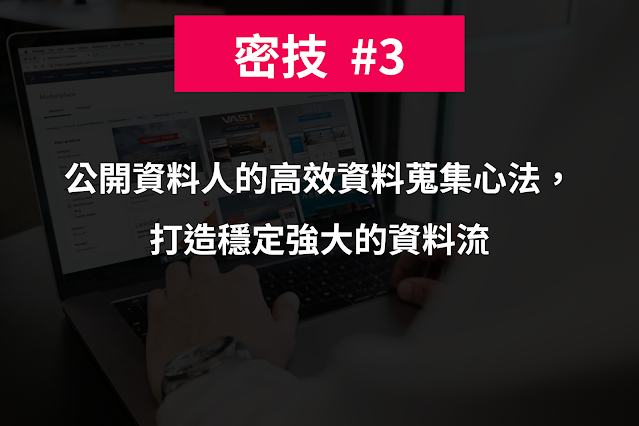
留言
張貼留言